Virtual Dressing Room
The PICTOFiT SDK allows your users to virtually try-on individual pieces, create looks and see how they fit & look on their avatar. Depending on the type of assets, this functionality is available in 2D and 3D. See the respective section about the PICTOFiT Content Service to learn how to create assets of your fashion products.
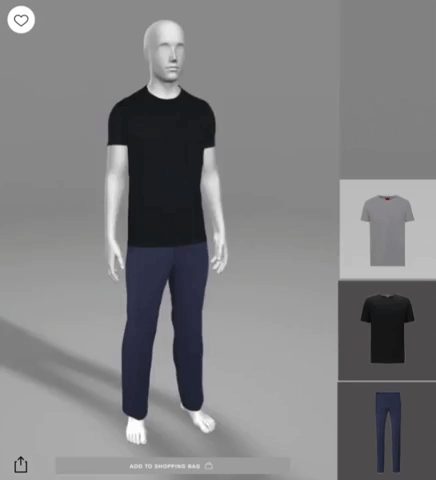
Introduction
In order to perform a virtual try-on you first need an avatar and one or more garments. You can also (optionally) customise the background/environment of your virtual dressing room through scenes.
Using these three components, you can conveniently integrate virtual try-on into your web application. The Pictofit.IVirtualDressingRoom
interface defines all required properties and methods to populate the dressing room with content and trigger the try-on, size recommendation and other related features. The example below shows how this is done in just a few lines of code. In the following sections, we explain the different steps involved.
let dressingRoom = Pictofit.VirtualDressingRoomFactory.createDressingRoom3D(viewer, computeServer);
dressingRoom.avatar = await dressingRoom.createAvatar(new Pictofit.StaticAsset("https://myserver.com/avatars/12354/"));
dressingRoom.garments = [
await dressingRoom.createGarment(new Pictofit.StaticAsset("https://myserver.com/garments/12354/")),
await dressingRoom.createGarment(new Pictofit.StaticAsset("https://myserver.com/garments/62356/"))
]
dressingRoom.scene = await dressingRoom.createScene(new Pictofit.StaticAsset("https://myserver.com/scenes/street/"));
dressingRoom.refresh();
Virtual Dressing Room
The VirtualDressingRoom (VDR) is the central compnent which provides the functionality to create an immersive virtual try-on experience with just a few lines of code. All available VDRs implement the same interface IVirtualDressingRoom
as well as the IAssetFactory
. The asset factory makes sure that you instantiate the correct types of garments, avatars, scenes and animations to match your chosen implementation. You still have to be careful about the underlying assets you are using to create them.
To create garments, avatars, scenes or animations, use the IAssetFactory
factory functions:
createAvatar(asset: IAsset): Promise<IAvatar>;
createGarment(asset: IAsset): Promise<IGarment>;
createScene(asset: IAsset): Promise<IScene>;
createAnimation(asset: IAsset): Promise<IAnimation>;
To set garments, avatar, scene or animation set the previously created instances to their respective properties:
get garments(): Array<IGarment> | null;
set garments(newGarments: Array<IGarment> | null);
get avatar(): IAvatar | null;
set avatar(newAvatar: IAvatar | null);
get scene(): IScene | null;
set scene(newScene: IScene | null);
get animation(): IAnimation | null;
set animation(newAnimation: IAnimation | null);
There can always only be one avatar, one scene and one animation. Setting more than one garment does increase try on computation times. We recommend to not use more then 3-4 garments simultaneously.
Setting any of these properties to null
is also possible if you want to only show the avatar for example after you already set some garments.
Some VDR implementation do place dependencies on some of these properties. For example a Parallax VDR does not allow to show an avatar without a scene.
Once you are done populating your VDR, call IVirtualDressingRoom.refresh()
. This will return a promise when the try-on is done updating / loading. Make sure to block your UI in the meantime. Otherwise the user might trigger another refresh while the dressing room is still loading.
The different implementations take care of all the logic and server communication for you. In order to instantiate the actual dressing room object we provide a Pictofit.VirtualDressingRoomFactory
. Depending on the available assets, we deliver concrete implementations for the following three scenarios:
2D
2D + Parallax
3D
Getting Started
To use the dressing room implementations, we also need to create a Pictofit.ComputeServer
and a Pictofit.WebViewer
instance.
const computeServer = new Pictofit.ComputeServer("https://myComputeServer", "myToken");
const webViewer = new Pictofit.WebViewer("canvas-id");
const dressingRoom = Pictofit.VirtualDressingRoomFactory.createDressingRoom2D(webViewer, computeServer);
The ComputeServer
objects handles the authentication & communication with the server. The WebViewer
is responsible for displaying and rendering the content in the user's browser.
To compute & show the try-on, we need to create a new Pictofit.IAvatar
instance and Pictofit.IGarment
instances. The dressing room interface provides factory methods for creating avatar, garment and scene objects. These methods ensure that the provided assets are of correct type (the 3D dressing room for example requires 3D avatar and 3D garment assets). At runtime, we check the assets and throw an exception if they don’t fit the required type.
dressingRoom.avatar = await dressingRoom.createAvatar(new Pictofit.StaticAsset("https://myserver.com/avatars/12354/"));
dressingRoom.garments = [await dressingRoom.createGarment(new Pictofit.StaticAsset("https://myserver.com/garments/12354/"))];
Please note that garments
is an array and that the order within the array defines the layering of the garments. This means that an item at index 1 of the array goes over an item at index 0 in the try-on.
To customize the background of a virtual try-on you create an object that implements the Pictofit.IScene
interface and simply set it on the dressing room.
dressingRoom.scene = await dressingRoom.createScene(new Pictofit.StaticAsset("https://myserver.com/scenes/street/"));
To to compute and render the virtual try-on we need to call the refresh(): Promise<void>
method.
dressingRoom.refresh();
Note:
If you use the same webviewer instance on the same page for different usecases make sure to release any existing resources within the dressing room by calling the cleanup(): Promise<void>
method.
dressingRoom.cleanup();
Avatars, Garments, Scenes and Animations
The creation of avatars, garments, scenes and animations follows the same principle and can be done conveniently using the Pictofit.IVirtualDressingRoom
implementations. To locate the resources of e.g. an avatar, you need to provide an object that implements the IAsset interface. The following section describes the different options in detail. In most cases, the Pictofit.StaticAsset
class will be the appropriate choice as it allows access to assets through a base URL.
For an avatar this could look like:
const avatar = await dressingRoom.createAvatar(new Pictofit.StaticAsset("https://myserver.com/avatars/12354/"));
For a garment this could be:
const garment = await dressingRoom.createGarment(new Pictofit.StaticAsset("https://myserver.com/garments/12354/"));
For a scene this could be:
const scene = await dressingRoom.createScene(new Pictofit.StaticAsset("https://myserver.com/scenes/street/"));
And for an animation this could be:
const animation = await dressingRoom.createAnimation(new Pictofit.StaticAsset("https://myserver.com/animations/default/"));
Size Recommendation and Visualisation
The PICTOFiT SDK allows you to compute the recommended size of a product for a certain avatar. It is very convenient and easy to use this feature. The Pictofit.IVirtualDressingRoom
provides appropriate methods for this. Have a look at the Size Recommendation & Fit Visualisation section for a detailed description.
Animations
Adding animations to your virtual dressing room will breathe life into otherwise static objects. This can be achieved by creating a Pictofit.IAnimation
instance and setting it onto the Pictofit.IVirtualDressingRoom
instance:
const animation = await dressingRoom.createAnimation(new Pictofit.StaticAsset("https://myserver.com/animations/default/"));
dressingRoom.animation = animation;
dressingRoom.refresh();
Not all assets support animations. If an avatar or one of the dressed garments does not have animation support all animations will be disabled.
Some VDR implementations like the Pictofit.VirtualDressingRoom2D
do not support animations at all.
Assets
Avatars, garments, scenes and animations are created from assets. An asset is basically a collection of individual components (files). In order to perform all kinds of computations optimally, the information of an avatar or garment is split up into multiple parts, its components, which are used individually instead of having everything saved in one single packet of data. When now instantiating for example a garment, we need to provide access to the respective data. The Pictofit.IAsset
interface defines the required methods. Please check out the page on Assets to learn more.